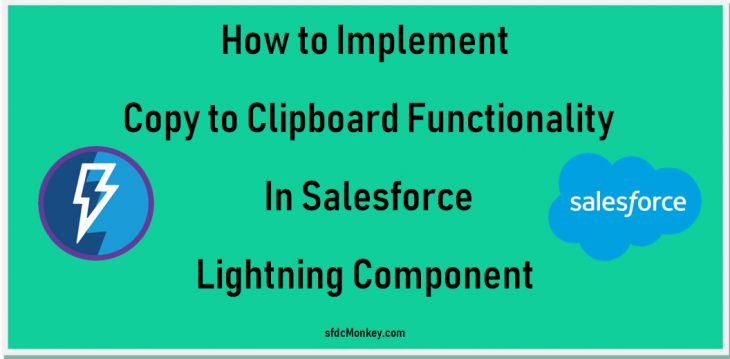
Copy To Clipboard Functionality In Salesforce Lightning Component
Hi guys, today in this post we are going to learn how we can implement ‘copy to clipboard‘ functionality in salesforce lightning component with client side JavaScript code. with this component you can copy text either from any input field or any hard-code text by clicking on button.
From developer console >> file >> new >> Lightning Component
Lightning Component : copyToClipboard.cmp
<!-- Source : sfdcmonkey.com Date : 1/23/2019 API : 44.0 --> <aura:component implements="flexipage:availableForAllPageTypes" access="global" > <div> <p aura:id="pId">quick brown fox jumps over the lazy dog</p> <lightning:button iconName="utility:copy_to_clipboard" onclick="{!c.copyHardcoreText}" label="Copy Text to clipboard" aura:id="btn1"/> </div> <div> <lightning:textarea value="hello i am textarea value" aura:id="inputF"/> <lightning:button iconName="utility:copy_to_clipboard" onclick="{!c.copyInputFieldValue}" label="Copy Text to clipboard" aura:id="btn2"/> </div> <div> <lightning:textarea value="" label="Paste Here (for testing)"/> </div> </aura:component>
JavaScript Controller : copyToClipboardController.js
({ copyHardcoreText : function(component, event, helper) { // get HTML hardcore value using aura:id var textForCopy = component.find('pId').getElement().innerHTML; // calling common helper class to copy selected text value helper.copyTextHelper(component,event,textForCopy); }, copyInputFieldValue : function(component, event, helper) { // get lightning:textarea field value using aura:id var textForCopy = component.find('inputF').get("v.value"); // calling common helper class to copy selected text value helper.copyTextHelper(component,event,textForCopy); }, })
JavaScript helper: copyToClipboardHelper.js
({ copyTextHelper : function(component,event,text) { // Create an hidden input var hiddenInput = document.createElement("input"); // passed text into the input hiddenInput.setAttribute("value", text); // Append the hiddenInput input to the body document.body.appendChild(hiddenInput); // select the content hiddenInput.select(); // Execute the copy command document.execCommand("copy"); // Remove the input from the body after copy text document.body.removeChild(hiddenInput); // store target button label value var orignalLabel = event.getSource().get("v.label"); // change button icon after copy text event.getSource().set("v.iconName" , 'utility:check'); // change button label with 'copied' after copy text event.getSource().set("v.label" , 'copied'); // set timeout to reset icon and label value after 700 milliseconds setTimeout(function(){ event.getSource().set("v.iconName" , 'utility:copy_to_clipboard'); event.getSource().set("v.label" , orignalLabel); }, 700); } })
- Check code comments.
TestApp :
From developer console >> file >> new >> Lightning Application
<aura:application extends="force:slds">
<c:copyToClipboard/>
<!-- here c: is org. default namespace prefix-->
</aura:application>
Output :
Other popular Post :
- How To Use jQuery DataTable Plugin In Salesforce Lightning Component -: Sample
- Powerful Lightning Datatable base component – Example Using Fieldset
- Custom Data Table With Inline Editing In Salesforce Lightning Component – Sample
- Add Delete Row Dynamic In Lightning Component : Sample Code
- Add Multiple Child Records to Parent Object With Lightning Component
Like our facebook page for new post updates. & Don’t forget to bookmark this site for your future reference.
if you have any suggestions or issue with it, you can post in comment box.
Happy Learning
One thought on “Copy To Clipboard Functionality In Salesforce Lightning Component”
This is works great. I am wondering if there is a way to use my own text and have line breaks in it. When I use the code below, the \t successfully makes a tab. However the \r\n ends up getting stripped out when it creates the hidden element on the document
to handle multiline